https://www.hackerrank.com/challenges/java-date-and-time/problem?isFullScreen=true
Java Date and Time | HackerRank
Print the day of a given date.
www.hackerrank.com
https://docs.oracle.com/javase/7/docs/api/constant-values.html#java.util.Calendar.DAY_OF_WEEK
Constant Field Values (Java Platform SE 7 )
docs.oracle.com
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.regex.*;
class Result {
/*
* Complete the 'findDay' function below.
*
* The function is expected to return a STRING.
* The function accepts following parameters:
* 1. INTEGER month
* 2. INTEGER day
* 3. INTEGER year
*/
/*
DAY_OF_WEEK
public static final int DAY_OF_WEEK
Field number for get and set indicating the day of the week. This field takes values SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, and SATURDAY.
SUNDAY 1
MONDAY 2
TUESDAY 3
WEDNESDAY 4
THURSDAY 5
FRIDAY 6
SATURDAY 7
*/
public static String findDay(int month, int day, int year) {
String result = "";
Calendar rightNow = Calendar.getInstance();
rightNow.set(year, month-1, day);
int dayOfWeek = rightNow.get(Calendar.DAY_OF_WEEK);
switch (dayOfWeek){
case 1 : result = "SUNDAY";
break;
case 2 : result = "MONDAY";
break;
case 3 : result = "TUESDAY";
break;
case 4 : result = "WEDNESDAY";
break;
case 5 : result = "THURSDAY";
break;
case 6 : result = "FRIDAY";
break;
case 7 : result = "SATURDAY";
break;
}
return result;
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
String[] firstMultipleInput = bufferedReader.readLine().replaceAll("\\s+$", "").split(" ");
int month = Integer.parseInt(firstMultipleInput[0]);
int day = Integer.parseInt(firstMultipleInput[1]);
int year = Integer.parseInt(firstMultipleInput[2]);
String res = Result.findDay(month, day, year);
bufferedWriter.write(res);
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
The Calendar class is an abstract class that provides methods for converting between a specific instant in time and a set of calendar fields such as YEAR, MONTH, DAY_OF_MONTH, HOUR, and so on, and for manipulating the calendar fields, such as getting the date of the next week.
You are given a date. You just need to write the method, , which returns the day on that date. To simplify your task, we have provided a portion of the code in the editor.
Example
The method should return as the day on that date.
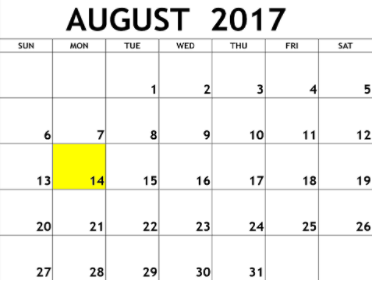
Function Description
Complete the findDay function in the editor below.
findDay has the following parameters:
- int: month
- int: day
- int: year
Returns
- string: the day of the week in capital letters
Input Format
A single line of input containing the space separated month, day and year, respectively, in format.
Constraints
Sample Input
08 05 2015
Sample Output
WEDNESDAY
Explanation
The day on August th was WEDNESDAY.
'HackerRank > JAVA' 카테고리의 다른 글
Java Substring (0) | 2024.01.16 |
---|---|
Java Strings Introduction (0) | 2024.01.16 |
Java Static Initializer Block (0) | 2024.01.12 |
Java Datatypes (0) | 2024.01.12 |
Java Loops II (0) | 2024.01.12 |